Courses
If you are absolutely new to Haskell, start with the Beginner crash course. This course is adapted from the six-hour beginner workshop we taught online for ZuriHac 2020. This is not a complete collection of everything you need to know about Haskell; it focuses on a small set of things that new programmers can learn in two days.
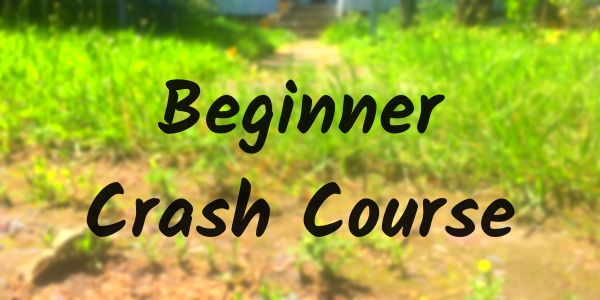
The Haskell Phrasebook is a free quick-start Haskell guide comprised of a sequence of small annotated programs. It provides a cursory overview of selected Haskell features, jumping-off points for further reading, and recommendations to help get you writing programs as soon as possible.
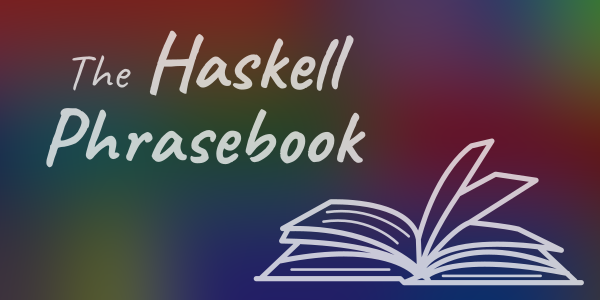
The course begins with two lessons on case expressions to ensure a solid foundation. From there, we write three functions for checking that inputs are valid passwords according to the rules of our system. The rest of the lessons iteratively expand on and refactor those functions into a small program that validates usernames and passwords, constructs a User if both are valid inputs, and returns pretty error messages if they are not. Along the way we learn about Monad and Applicative and how they are similar and different and how to use types to rethink our solutions to problems.
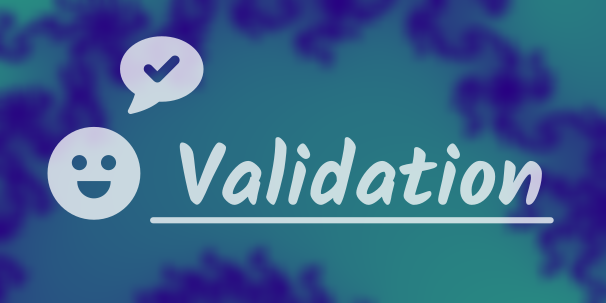
This series gives a detailed explanation of the two most basic functor typeclasses, including examples of usage, and their laws. It also introduces using the ‘hedgehog’ library for property testing.
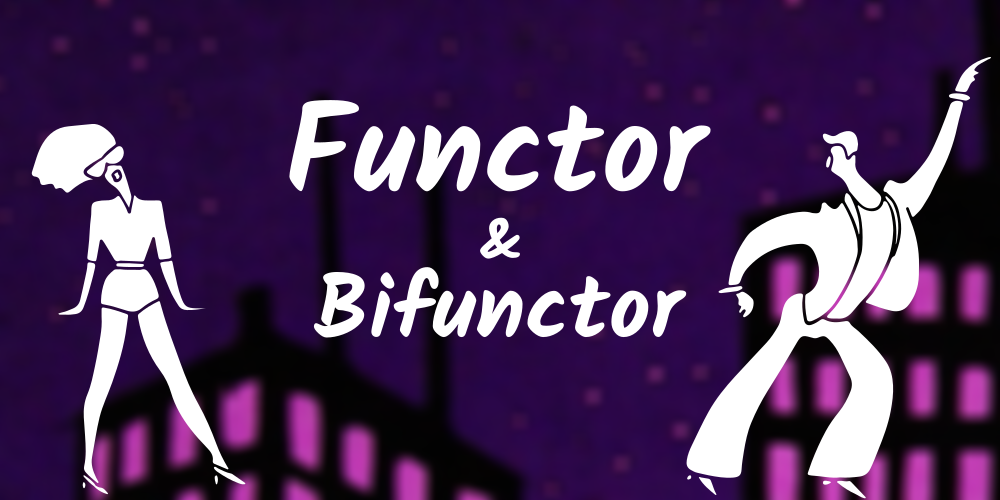
This series covers the ‘Applicative’ typeclass. After thoroughly treating several practical examples and some important applicative functors, we look at the laws of the class and property testing them with ‘hedgehog’.
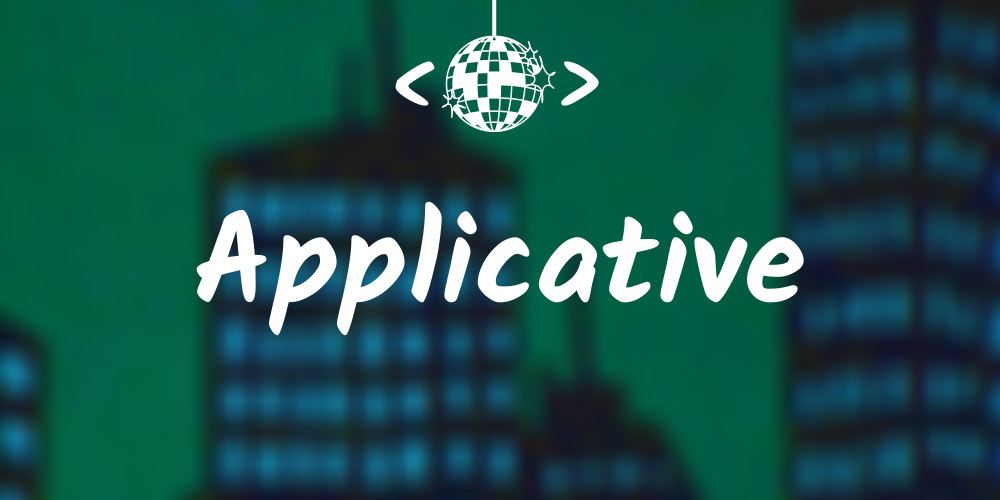
The Haskell REPL, GHCi, is a great interactive development tool with a lot of features. This series explains some aspects of GHCi that are not always obvious and brings some of those features to light.
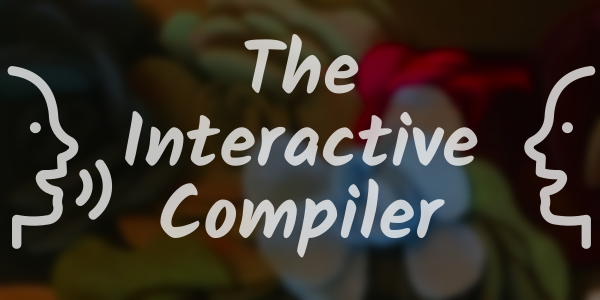
This course uses Haskell to explore what a web server is, starting from how we use sockets and the network library, and moving toward a foundation for higher-level libraries for building web applications. On the way, you will learn: what is a socket; how to read the HTTP specification; the difference between a strict ByteString, a lazy ByteString, and a byte string Builder; what “chunked transfer coding” means in HTTP, and what that has to do with lazy I/O; how to use Attoparsec to parse an HTTP request; how to use the Pipes library to parse streaming input.
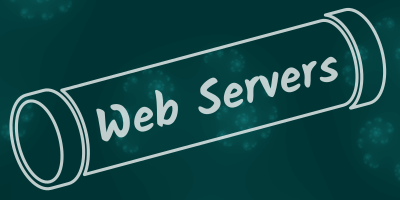
GHC Haskell has an overwhelming number of language extensions. Some are well documented, others are sparsely documented; some are common, others are exotic. It can be difficult terrain to navigate. Most programs can be written in vanilla Haskell with no extensions enabled. The extensions do not change the languages or its semantics. They are compiler extensions and change the way the compiler does its job, the way it reads the code. Hence, if you are using a compiler other than GHC (or are using a very old version of GHC), you may not have access to any of these extensions or only to some subset. Here we covers the extensions we think are most likely to be useful and helpful to the Haskell beginner or early intermediate as well as a few having to do with issues that crop up whether you’re ready for them or not. Each has a link to a longer article about it, describing it in more detail.
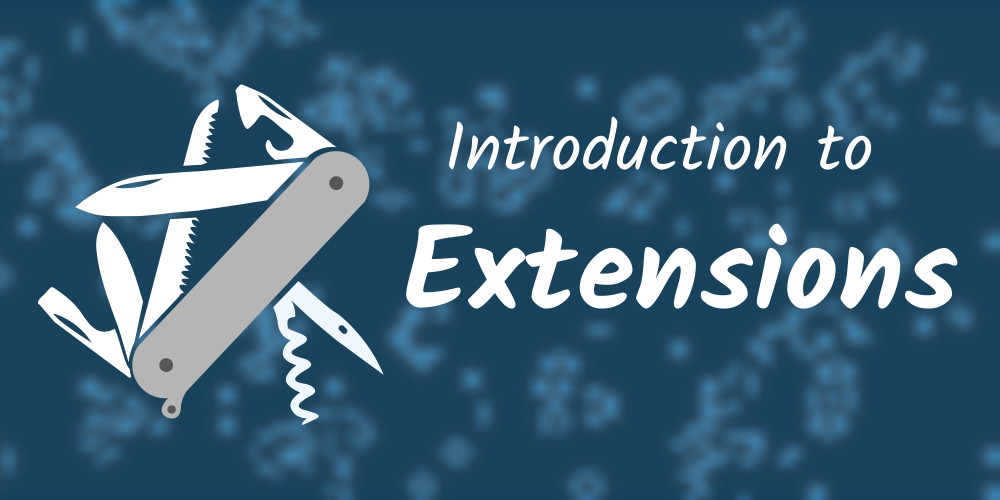
Four years later, we revisit a CSV processing project to think about what we might have done differently if we were writing it today. Could it be written more clearly? More efficiently? With other parsing libraries? Are there other interesting things we could learn from the data? Come join us on a trip through the design space and down memory lane.
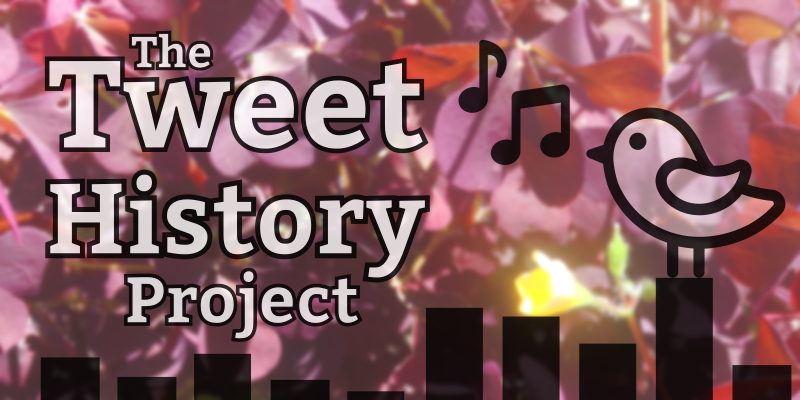
Nix is a general-purpose build tool, like a modern reinvention of Make that uses file hashes instead of timestamps. As Haskell fans we love it because the configuration language, a (mostly) pure lazy lambda calculus, has a familiar feel.
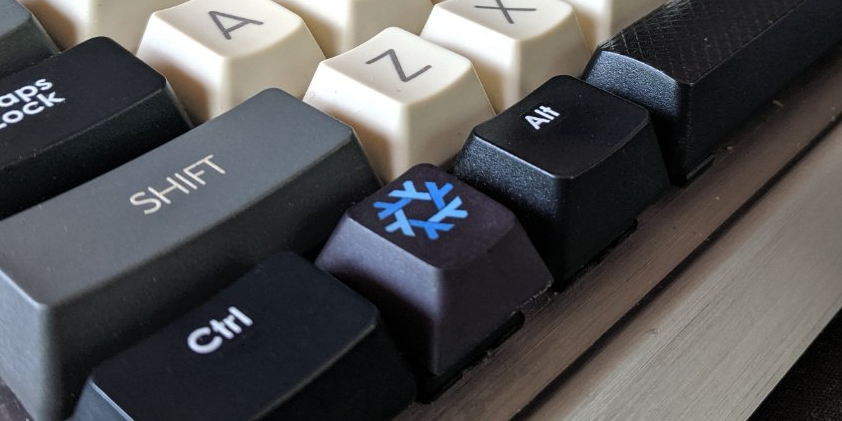
A Python iterator is a sort of sequence that, unlike a list, computes each subsequent value only as it is needed rather than computing the entire list all at once. In simple cases, Haskell’s list type (
[]
) fulfills a similar role. This series compares Python iterators to Haskell’s list datatype, taking a deep dive into the itertools
library and the corresponding functions in the Haskell standard library.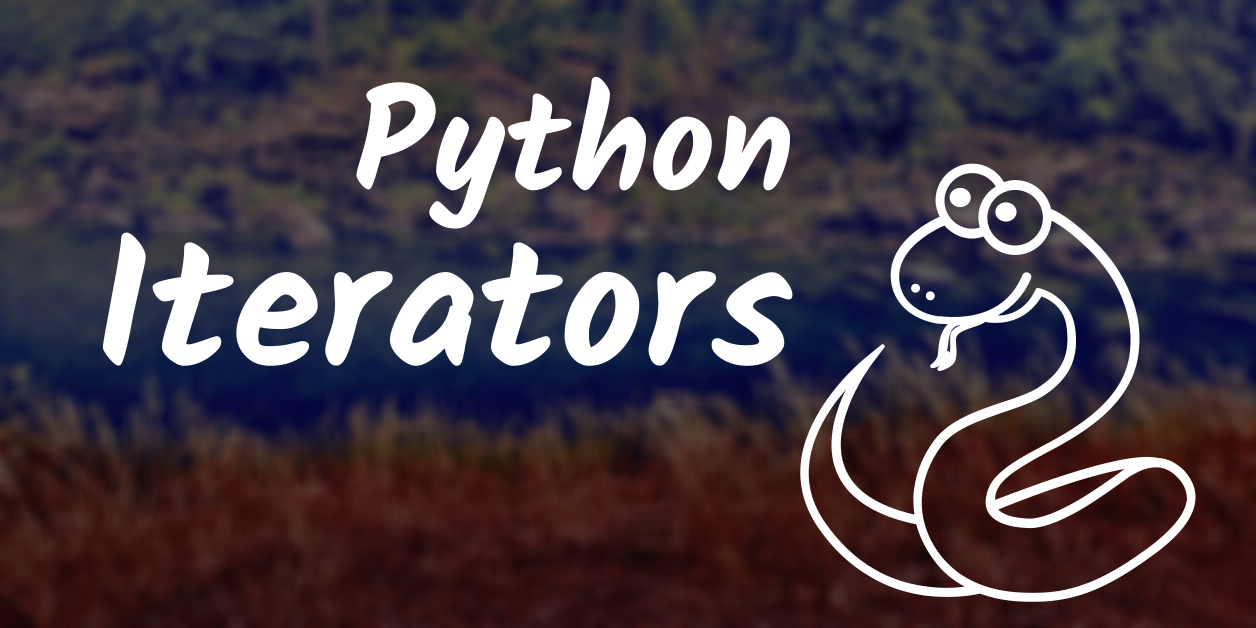